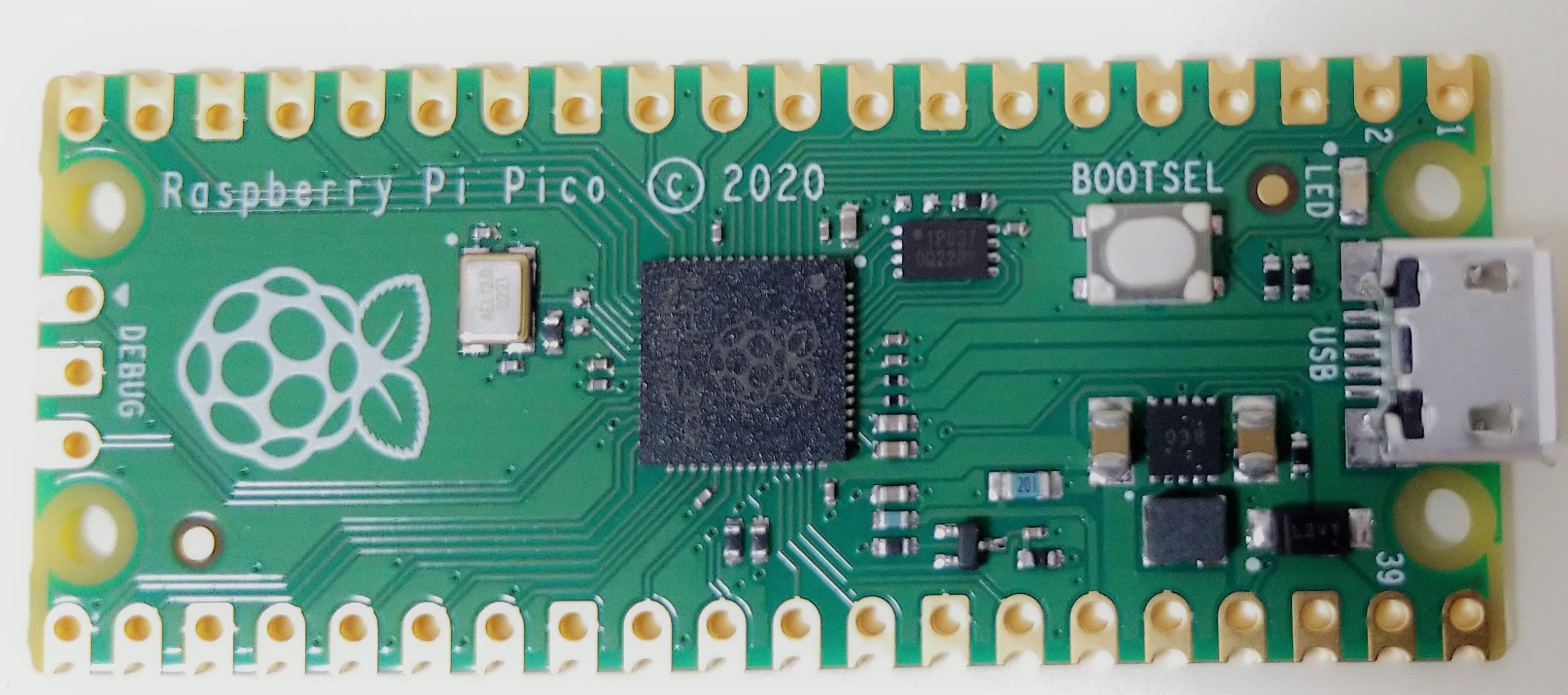
I2C on the Raspberry Pi Pico
The RP2040 has two independent channels of I2C controllers (I2C0 / I2C1), which are available on the Raspberry Pi Pico from GP[0] to GP[27] pins. In this article, we tried to use this feature to read and write the digital temperature sensor and EEPROM.Connecting the TMP102 digital temperature sensor module
The TMP102 device is a high-precision digital temperature sensor made by TI, with a built-in 12-bit ADC, a minimum resolution of 0.0625°C, and an accuracy of ±0.5°C without calibration or signal conditioning by external components.Here, we will use a SparkFun modulewith this temperature sensor IC and peripheral components (I2C pull-up resistor, etc.) to connect to a Raspberry pi pico.
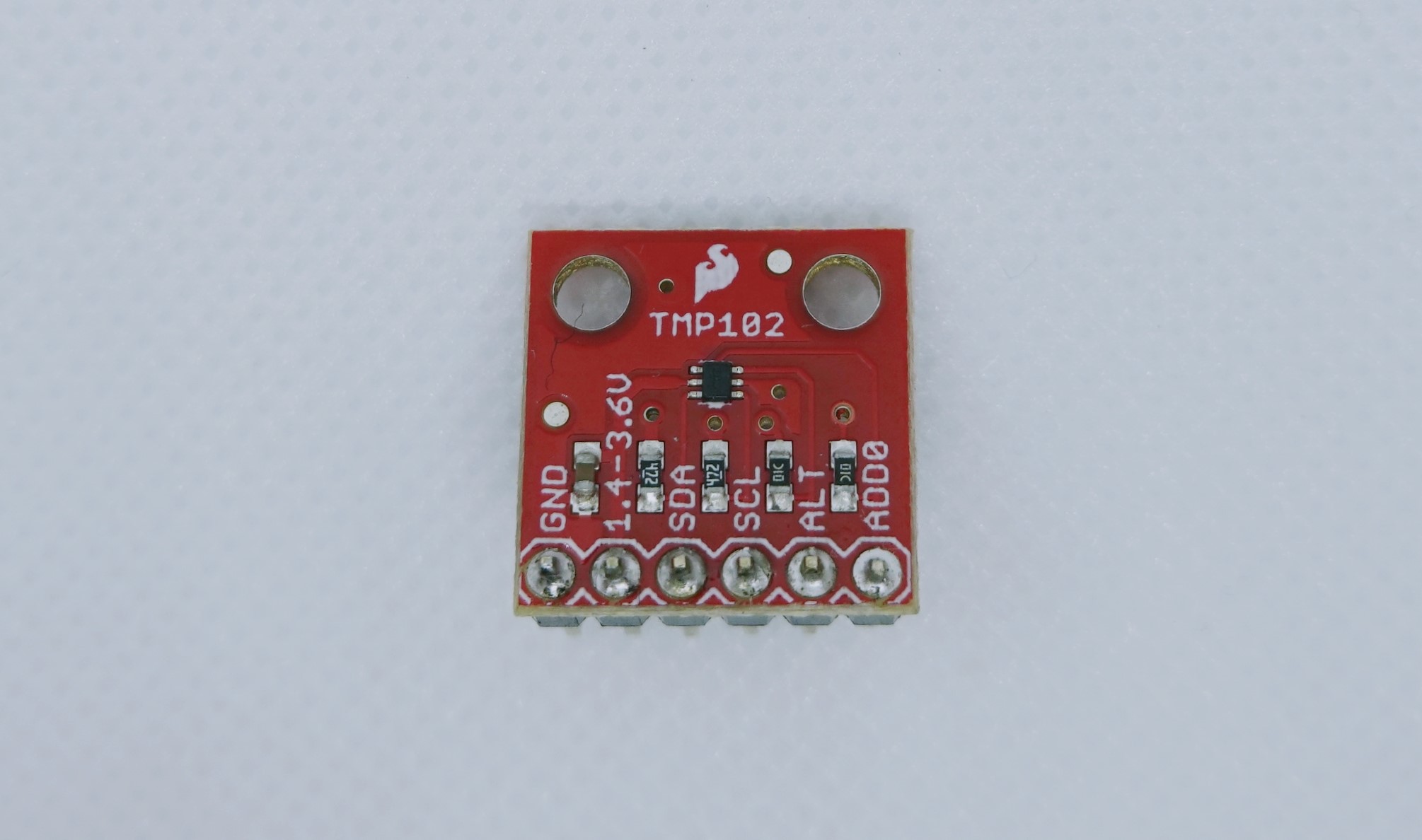
Hardware connection
The wiring between the Raspberry Pi Pico I/O terminals and the TMP102 module is shown below, and the I2C pull-up resistor and other necessary components are already mounted on the module board. The I2C slave address can be changed by the jumper setting on the module board, but the default address is 0x48.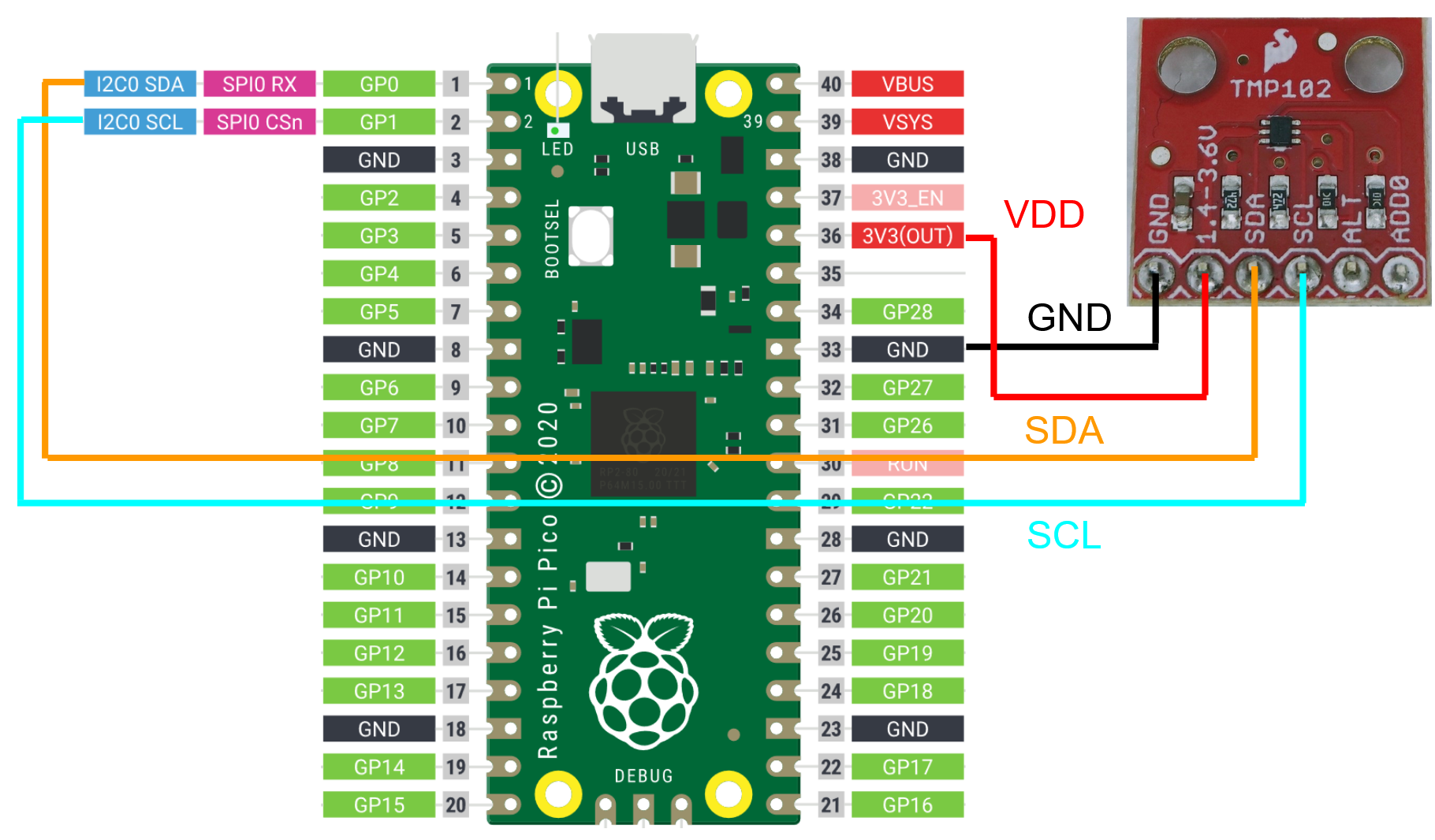
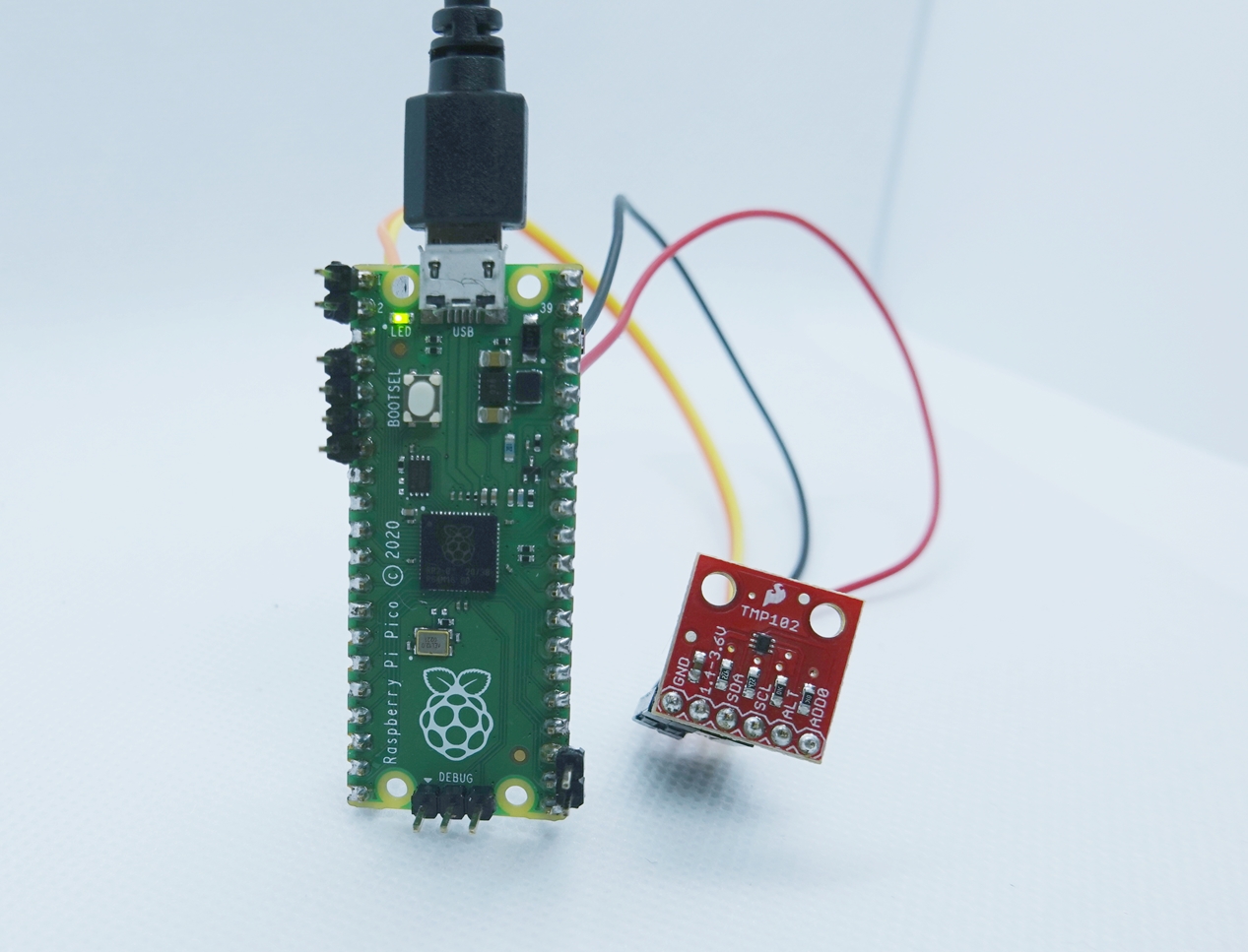
Software (Micro Python code)
As before, we will use Micro Python. Referring to the documentation of the MicroPython library and checking the examples of the I2C class used for the 2-wire serial protocol (I2C).# I2C configuration (GP0 pin is used as SDA, GP1 pin is used as SCL, and the clock is 100 kHz) i2c = I2C(0, scl=Pin(1), sda=Pin(0), freq=100000) # Scan for the address of the I2C device addr = i2c.scan() # Read 2 bytes from the device at slave address 0x48 data = i2c.readfrom(0x48, 2) # Write 2 bytes from memory address 0x02 at slave address 0x50 i2c.writeto_mem(0x50, 0x02, b'x10') # Read 3 bytes starting from memory address 0x08 at slave address 0x50 i2c.readfrom_mem(0x50, 0x08, 3)
from machine import Pin, I2C import time i2c = I2C(0, scl=Pin(1), sda=Pin(0), freq=100000) addr = i2c.scan() print( "address is :" + str(addr) ) for i in range(100): data = [] data = i2c.readfrom(0x48, 2) intdata = int.from_bytes(data, 'big') tmp = intdata >> 4 print(tmp * 0.0625) time.sleep(1)
Result
The result and the waveform during I2C reading are shown below.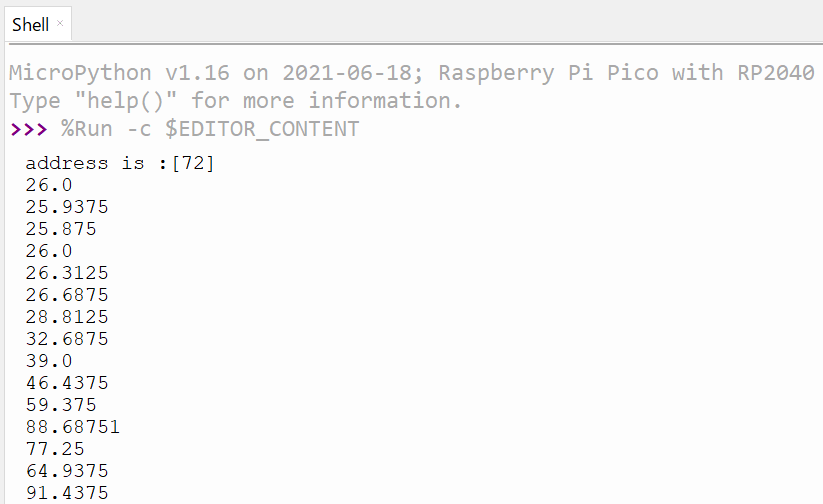
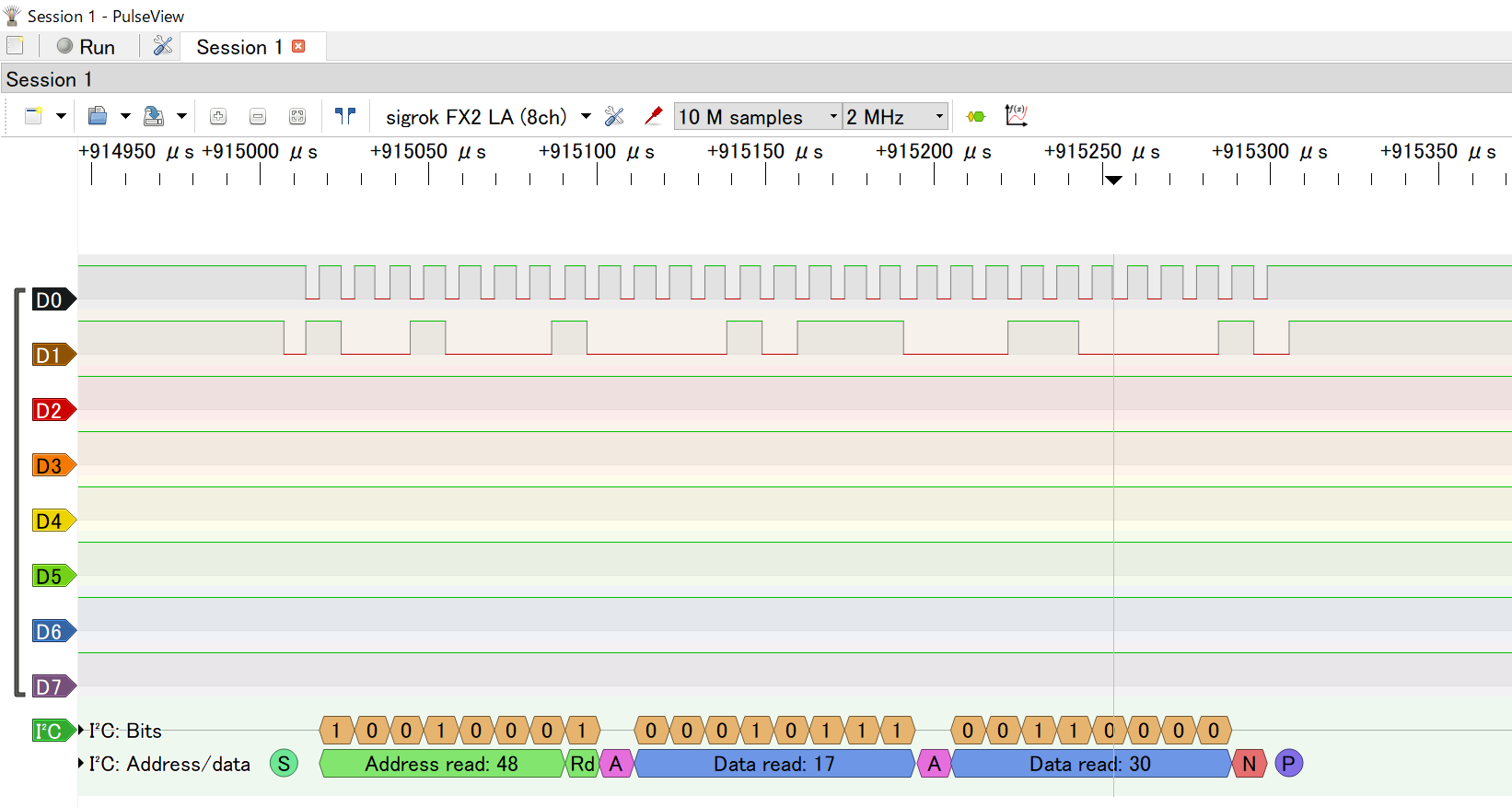
Connecting EEPROM
Next, let’s try to connect the serial EEPROM with I2C interface. The device to be used is the BR24L32 device manufactured by ROHM. (The picture below shows the device mounted on the pitch conversion board.)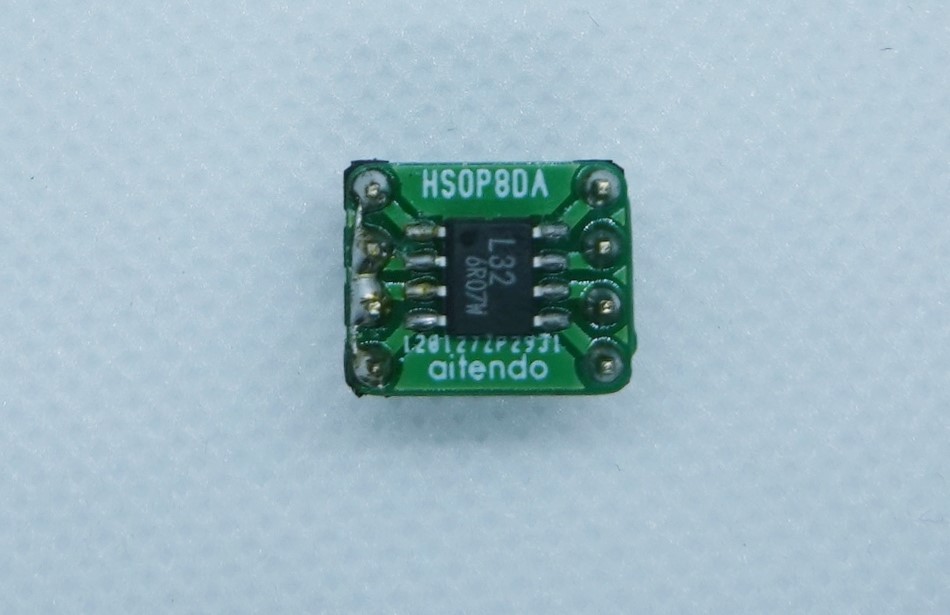
Hardware connection
In addition to the I2C, power, and GND pins, the /WP write protection control pin is connected to GP2 on the Raspberry Pi Pico. The I2C slave address selection pins A0 to A2 are connected to GND, and the slave address is 0x50.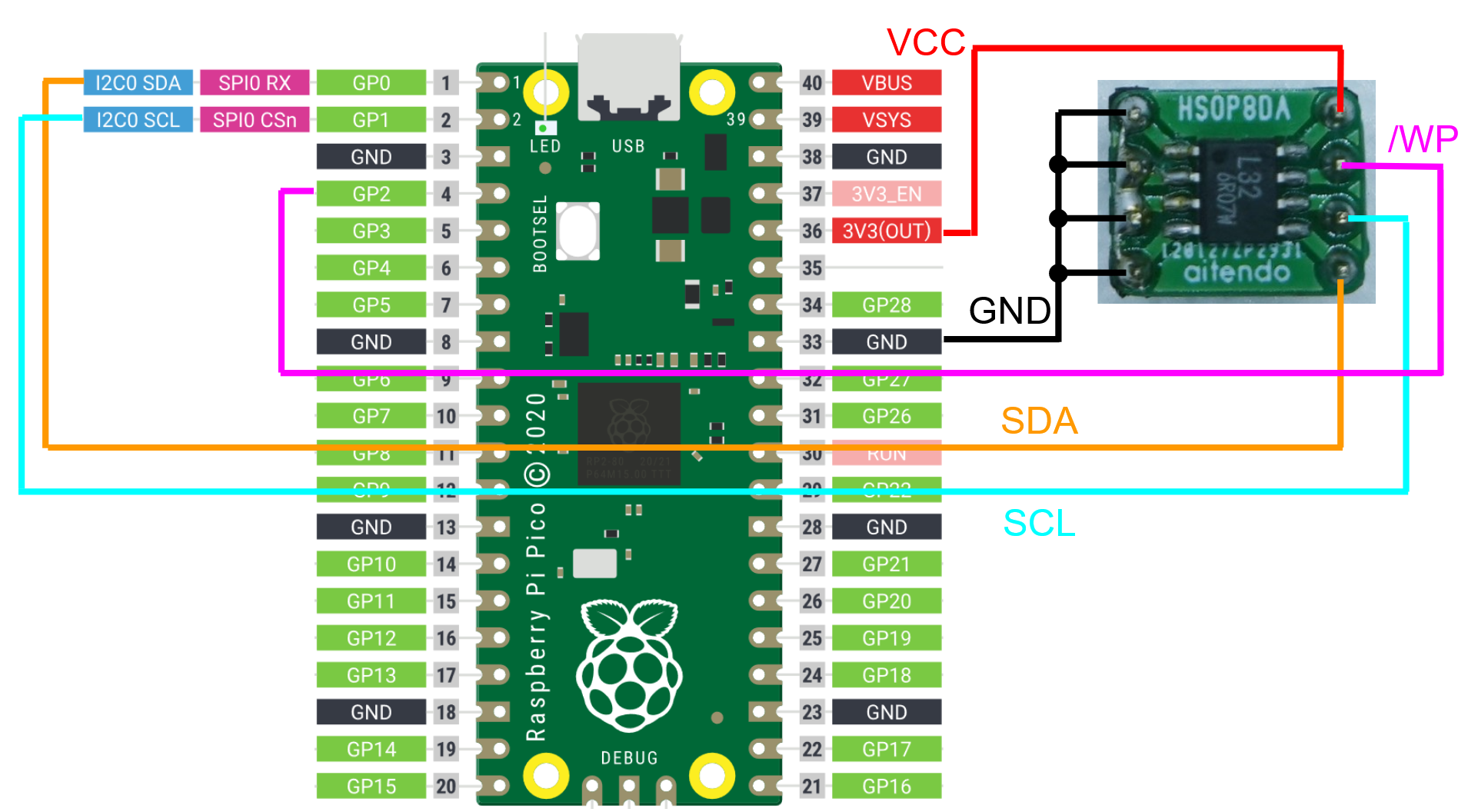
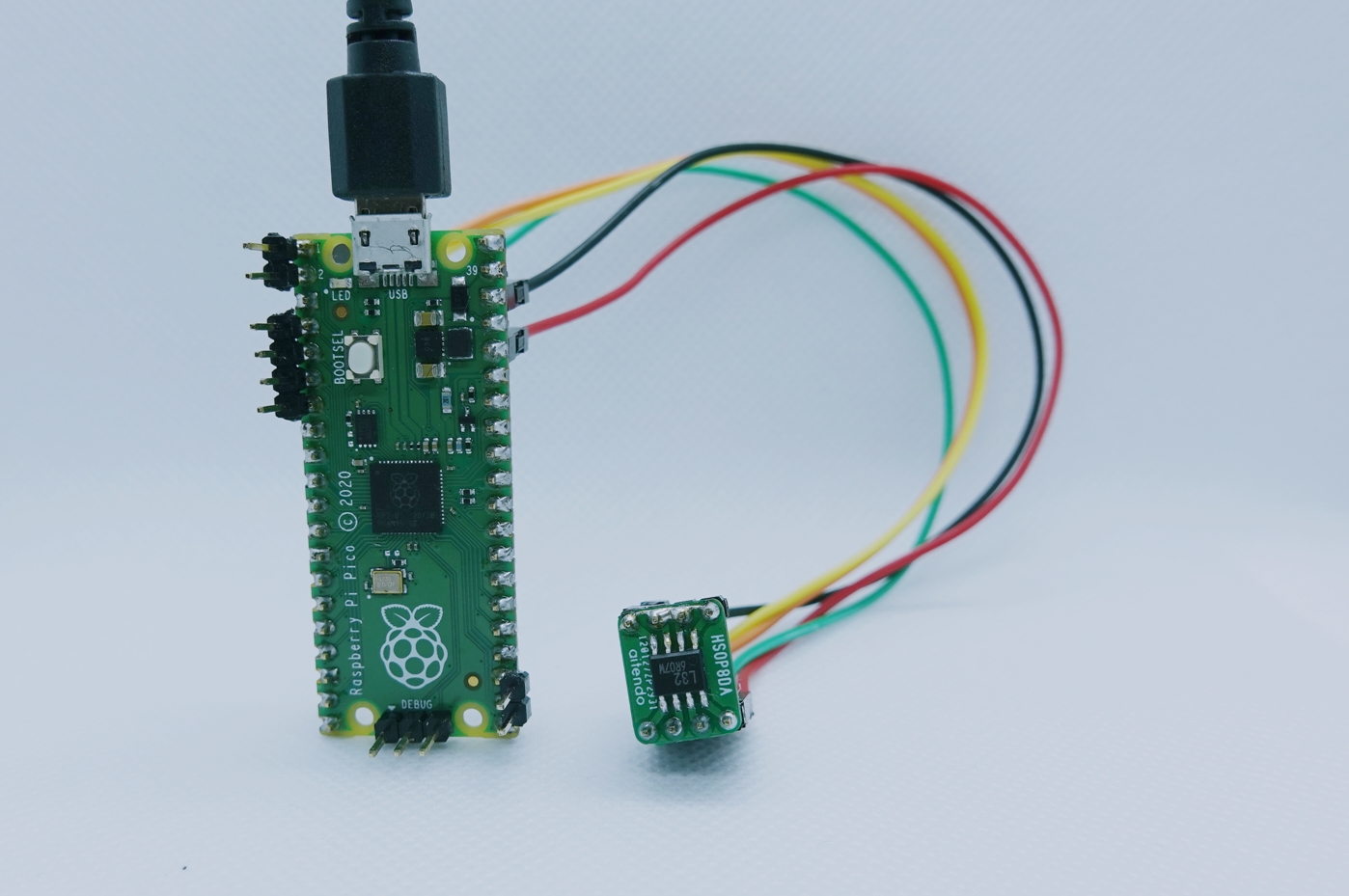
Software (Micro Python code)
The i2c.writeto_mem and i2c.readfrom_mem functions are used to write to and read from a memory address.from machine import Pin, I2C import time i2c = I2C(0, scl=Pin(1), sda=Pin(0), freq=100000) wp = Pin(2, Pin.OUT) addr = i2c.scan() print( "address is :" + str(addr) ) buff = bytearray(8) buff = bytes([0x00, 0x11, 0x22, 0x44, 0x88, 0x55, 0xAA, 0xFF]) #buff = bytes([0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00]) wp.value(0) # Read 8 bytes from memory address 0x0F00 data_r = i2c.readfrom_mem(int(addr[0]), 0x0F00, 8, addrsize=16) time.sleep_ms(5) for i in range(8): print(hex(data_r[i])) # Write 8 bytes from memory address 0x0F00 i2c.writeto_mem(int(addr[0]), 0x0F00, buff, addrsize=16) time.sleep_ms(5) print("----------") # Read 8 bytes from memory address 0x0F00 data_r = i2c.readfrom_mem(int(addr[0]), 0x0F00, 8, addrsize=16) time.sleep_ms(5) for i in range(8): print(hex(data_r[i]))
Result
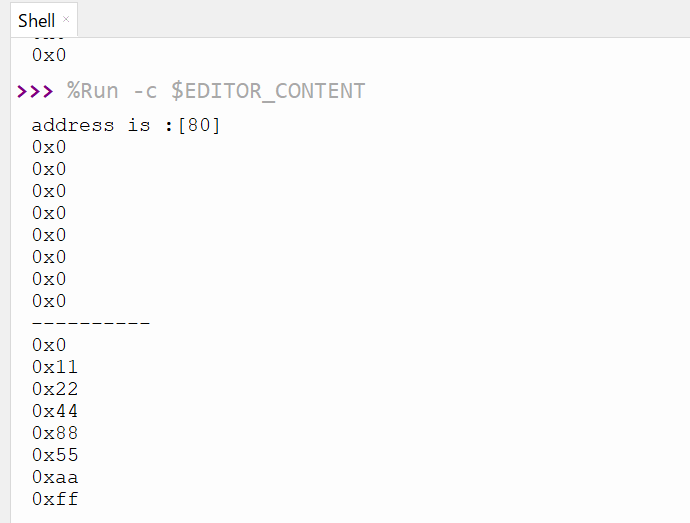
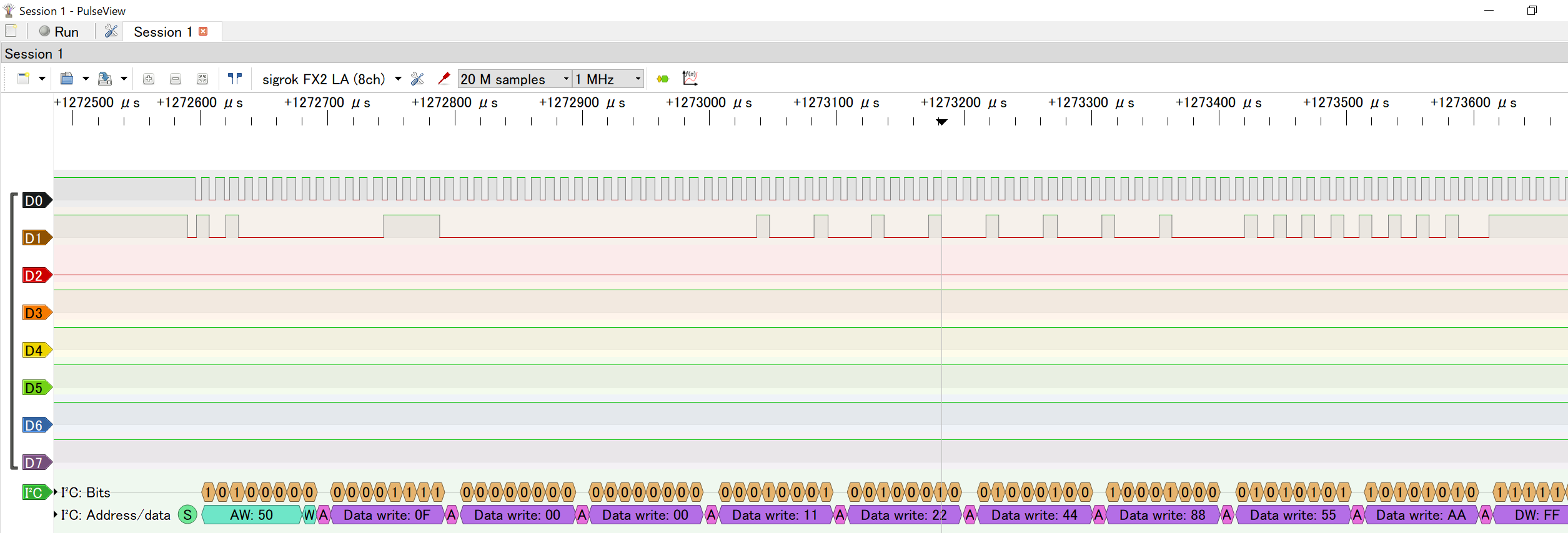
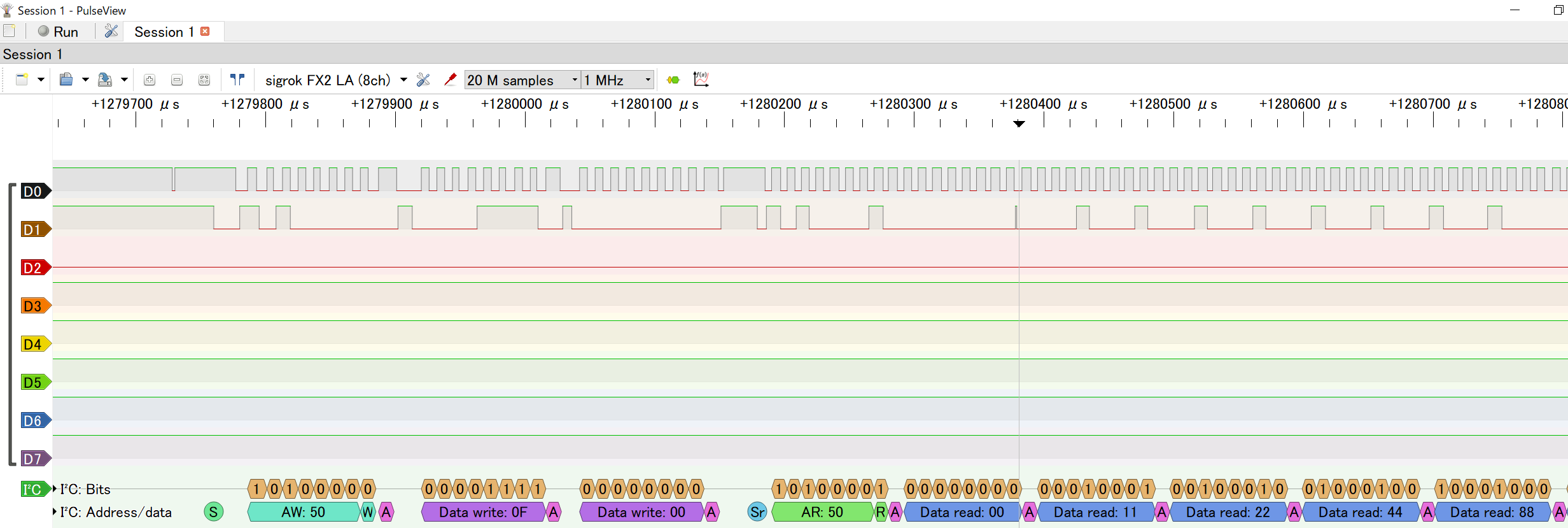
References
TI’s High Precision Digital Temperature Sensor TMP102SparkFun TMP102 Module
MicroPython Library Documentation
ROHM BR24